How To Generate TypeScript Types For Any GraphQL API
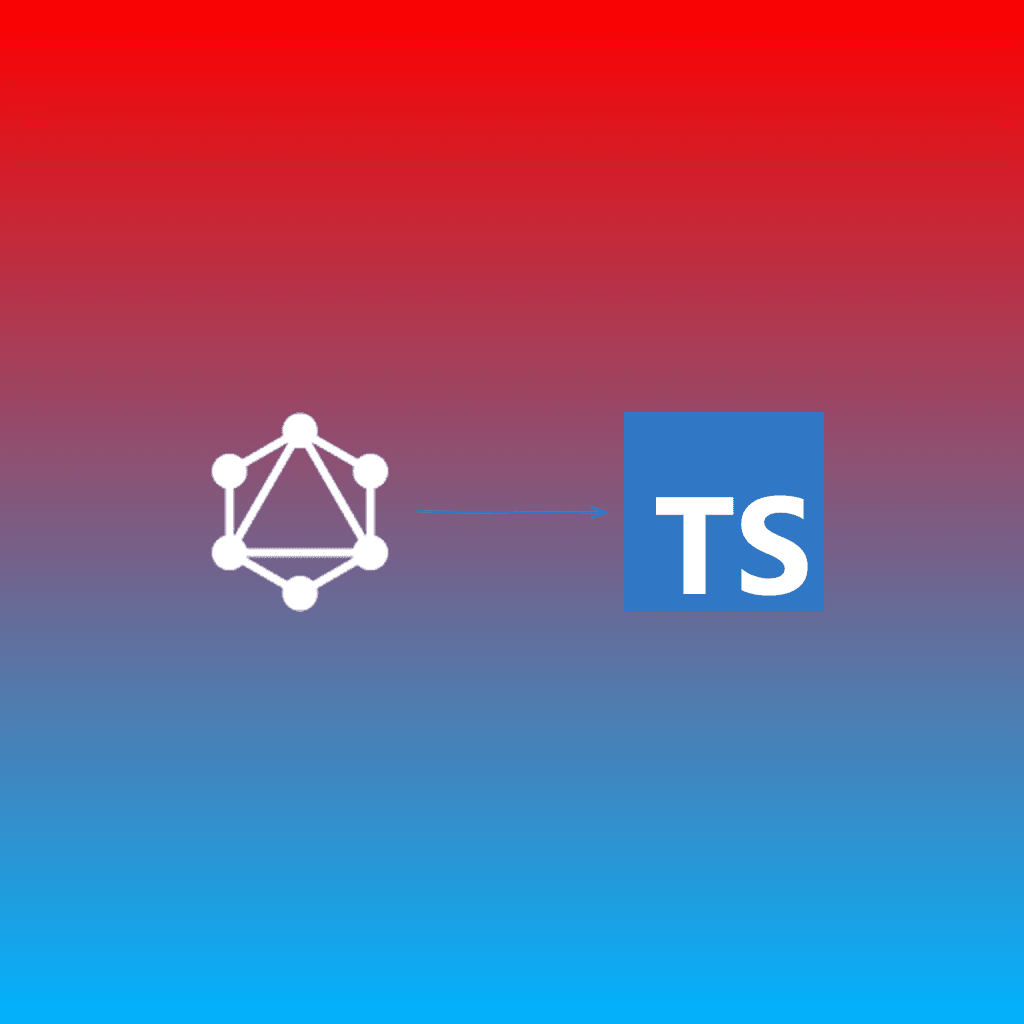
Introduction
(Type) Safety First!
Building robust frontend and backend solutions requires a reliable source of truth for your data. Whether you’re working on a simple marketing website with modular components or a complex e-commerce platform integrating Salesforce and Stripe APIs, type safety is key. It reduces bugs, simplifies collaboration, and ensures consistency across your application.
Getting Started
Prerequisites
- A Next JS App (detailed in Step 1)
- A Working GraphQL API / Backend
- I will be using a WPGraphQL instance on a basic WordPress install.
- You can use whatever you’d like! This should hold true for all options as long as the GraphQL API allows introspection.
Step 1: Clone an empty Next.js repo
With your package manager of choice create a new next js project. I’ll be using Bun
1bun create next-app@latest [your-project-name] --ts
Step 2: Install Dev Dependencies
I am using GraphQL Codegen to build out the types in the command line.
- @graphql-codegen/cli
- @graphql-codegen/typescript-operations
- @graphql-codegen/typescript-react-apollo
- @graphql-codegen/typescript-nhost (If you need to add headers and other options for auth.)
- child_process
- dotenv
1bun install -D @graphql-codegen/typescript-nhost @graphql-codegen/typescript-react-apollo @graphql-codegen/typescript-operations @graphql-codegen/cli child_process dotenv
Your package.json
should look something like this:
1{
2 "name": "next-graphql-types",
3 "version": "0.1.0",
4 "private": true,
5 "scripts": {
6 "dev": "next dev --turbopack",
7 "build": "next build",
8 "start": "next start",
9 "lint": "next lint"
10 },
11 "dependencies": {
12 "react": "^19.0.0",
13 "react-dom": "^19.0.0",
14 "next": "15.1.6"
15 },
16 "devDependencies": {
17 "@eslint/eslintrc": "^3",
18 "@graphql-codegen/cli": "^5.0.3",
19 "@graphql-codegen/typescript-nhost": "^0.0.2",
20 "@graphql-codegen/typescript-operations": "^4.4.0",
21 "@graphql-codegen/typescript-react-apollo": "^4.3.2",
22 "@types/node": "^20",
23 "@types/react": "^19",
24 "@types/react-dom": "^19",
25 "child_process": "^1.0.2",
26 "dotenv": "^16.4.7",
27 "eslint": "^9",
28 "eslint-config-next": "15.1.6",
29 "postcss": "^8",
30 "tailwindcss": "^3.4.1",
31 "typescript": "^5"
32 }
33}
34
Step 3: Create Generation Files
Create three new files below at the root of your project.
1schema:
2 - ${NEXT_PUBLIC_WORDPRESS_URL}/index.php?graphql: # This will be your graphql endpoint
3 headers:
4 Origin: https://localhost:3000 # Add Headers or any other options as necessary
5generates:
6 ./types/wp.ts: # Will generate a file at this location
7 plugins: # specialized plugins if needed
8 - 'typescript'
9 - 'typescript-operations'
10 - 'typescript-react-apollo'
11 - 'typescript-nhost'
12 config:
13 withRefetchFn: true
Step 4: Modify package.json with new generate commands
Add within the scripts section.
1{
2 "scripts": {
3 "generate": "bun generate.js"
4 }
5}
Step 5: Run the command and start using your generated types!
1bun generate
Type Safety Achieved
Start using your types
types/wp
that has all the types from your GraphQL queries and mutations.
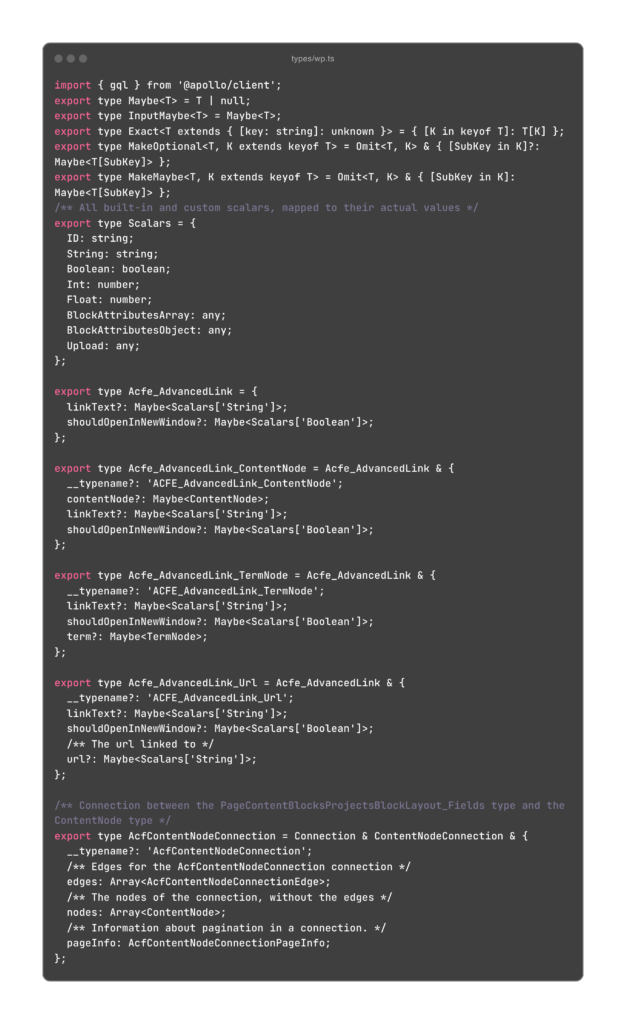
Here are some ways these types can be utilized
1import { PageContentBlocksCardsBlockLayout } from "@workspace/ui/types/wp"; // Importing Generated Types
2import PopupCards from "@workspace/ui/components/card/popup-cards";
3
4// Custom type intersection so I can add custom internal build properties while maintaining types safety with the backend.
5type CardsBlockProps = PageContentBlocksCardsBlockLayout & {
6 className?: string;
7};
8
9
10export const CardsBlock = ({
11 title,
12 content,
13 cards,
14 className,
15}: CardsBlockProps) => {
16 return (
17 <div
18 className={`cards-block ${className} py-4 md:py-16 flex flex-col gap-[48px]`}
19 >
20 <div className="flex gap-2 md:gap-6 flex-col md:flex-row justify-between items-start md:items-end">
21 {title && (
22 <h2 className="text-2xl md:text-5xl font-bold md:min-w-[50%] balanced">
23 {title}
24 </h2>
25 )}
26 {content && (
27 <div
28 className="text-sm text-foreground/60"
29 dangerouslySetInnerHTML={{ __html: content }}
30 />
31 )}
32 </div>
33 <PopupCards cards={cards} />
34 </div>
35 );
36};
37
38export default CardsBlock;
Conclusion
Type safety streamlines development, reduces errors, and ensures your application is maintainable and scalable. By generating TypeScript types for your GraphQL API, you align your frontend and backend seamlessly, allowing you to focus on delivering outstanding user experiences.
To further explore this synergy, consider experimenting with custom GraphQL configurations, integrating advanced plugins, or optimizing your code generation process. If you’d like guidance, feel free to reach out!